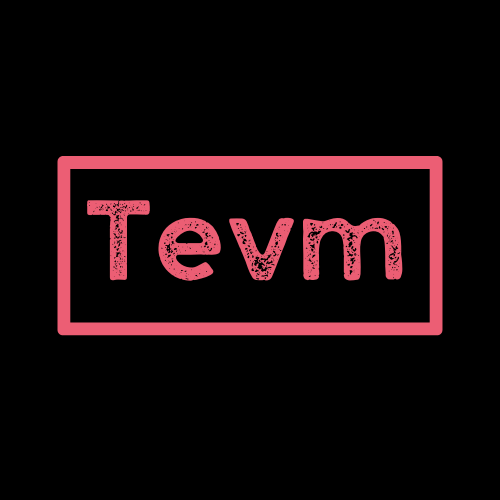
An Ethereum Node built to run in Browser, Bun, Deno, and Node.js
// @noErrors
// Runs in browsers, Node.js, Deno, Bun and beyond
// Zero native dependencies
import { createMemoryClient } from "tevm";
// Direct Solidity imports with the Tevm Bundler
import { ComplexSimulation } from "../contracts/ComplexSimulation.s.sol";
const client = createMemoryClient();
// Use powerful TypeScript native APIs or use the viem APIs you already know
const {
data,
error,
logs,
createdAddresses,
executionGasUsed,
l1Fee,
trace,
accessList,
txHash,
} = await client.tevmContract({
deployedBytecode: ComplexSimulation.deployedBytecode,
...ComplexSimulation.read.simulate(2n, "arg2"),
createTrace: true,
createAccessList: true,
createTransaction: true,
throwOnFail: false,
onStep: (step, next) => {
console.log(step.opcode);
next?.();
},
});
Up to 10x Faster Than Remote Calls
Execute transactions locally without network roundtrips. Instant gas estimates for responsive dApps.
Performance Benefits →Import Solidity Files Directly
Use .sol files as native ES modules with full TypeScript type safety.
Bundler Integration →Fork Any Chain
Create instant local copies of Ethereum, Optimism, or any EVM chain. Use against real contracts and state without external tools like Anvil or Hardhat.
Forking Guide →EVM Execution Hooks
Plug into EVM execution at the opcode level. Build advanced tools like debuggers, tracers, or custom gas profilers.
EVM Hooks Guide →Works With Tools You Know
Native integration with viem and full compatibility with ethers.js, wagmi, and any EIP-1193 library.
Library Support →"If you're building a blockchain application on the web, I'm almost certain there is a use case for Tevm. It might change everything, and at worst it would most likely improve your devX."
polarzero
@0xpolarzero
Turn Solidity into TypeScript
The Tevm Bundler lets you import Solidity contracts directly into your JavaScript or TypeScript code. No more copying ABIs or managing contract artifacts manually—just import your .sol files and get full type safety, auto-completion, and easy contract interaction.
// @noErrors
// Import Solidity contracts directly
import { ERC20 } from '@openzeppelin/contracts/token/ERC20/ERC20.sol'
// Use with full TypeScript support
const tokenContract = ERC20.withAddress('0x123...')
// Type-safe contract methods
const balance = await client.readContract(
tokenContract.read.balanceOf('0x456...')
)
// Coming soon: Import contracts directly from any chain
import { WETH } from 'caip10:eip155:1:0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2'
// Coming soon: Write Solidity inline
import { sol } from 'tevm'
const { abi, bytecode } = sol`
pragma solidity ^0.8.19;
contract Counter {
uint256 private count = 0;
function increment() public {
count += 1;
}
function getCount() public view returns (uint256) {
return count;
}
}
`
Here's our documentation as plain text for providing context to LLM systems